Hi, Iβm a Senior Software Engineer with the ΒιΆΉΤΌΕΔ Media Playout team. We are responsible for producing and maintaining the
Standard Media Player
The Standard Media Player is built on two core technologies, Adobe Flash and HTML5, and uses a decision engine to embed the appropriate client based on platform and device - for example the SMP Flash player is served to desktop and laptop computers, whilst the SMP HTML player is embedded on iOS devices. For desktop, Flash currently remains the primary method of delivering media playback to our users and this presents us with several challenges when making our services accessible to the widest range of users.
Accessibility and Flash
With the rise of HTML5 most browsers and operating systems provide good integration for accessibility (screen reader support, tab controls etc) however Flash has always been problematic and requires a lot more custom code in order to meet basic accessibility requirements. The launch of SMP allowed us to completely rebuild our accessibility implementation and ensure the player was designed and coded with the in mind.
The nature of Flash has always presented a challenge for users of assistive technologies. There are a number of technological and design considerations that must be taken into account when building accessible Flash content:
- A Flash movie consists of dynamic, vector-based graphics most of which are not identifiable to screen reader technologies in the same way a static HTML tag is
- Components are not always laid out in a logical or obvious fashion when compared to HTML
- Content in a Flash movie usually updates or animates, a visually impaired user may not be aware of this change
- Browser support for Flash varies massively between player and OS versions
- Screen reader and other assistive technologies have varying levels of support for Flash, in some cases none
- Flash is a browser-based plugin; OS accessibility settings such as colour contrasts will not be applied to Flash content
- Flash security restrictions prevent accessible keys working in full screen mode
For this post Iβm going to talk about how we addressed two fundamental accessibility requirements when building the Standard Media Player for Flash, keyboard controls and screen reader support.
Keyboard Controls
ΒιΆΉΤΌΕΔ accessibility guidelines, in keeping with existing W3C guidelines, state that all ΒιΆΉΤΌΕΔ websites and services must be
This covers a few basic concepts:
1. a user must be able to tab forwards and backwards into an interactive component
2. the component must have a clear focused state to indicate where the user is focused
3. the component must have a shortcut key where logical
4. if the component is a button then it must be activated with either the space or enter key
TabIndex vs Custom implementation
Flash already has basic tabbing functionality built in to allow display objects to be accessed via the keyboard. By setting the tabEnabled and tabIndex properties against a DisplayObject instance you are inserting it into the tab order of the movie and letting Flash player do the work when it comes to tab key events and moving focus between objects.
This approach to keyboard controls works well with a Flash movie embedded in page (with the stage.displayState property set to normal) and is the option many developers end up using as it requires very little custom code to implement. The problem with using the default tabbing functionality comes when we enter full screen. Flash security restrictions for full screen mode block most keyboard interactions including enter, space and other shortcut keys, which renders most buttons and interactive components useless. This obviously causes problems for keyboard users wanting to operate video in full screen mode.
To address the tabbing problems in SMP we decided that manually controlling the tab index in code was preferable. It not only allowed users to control playback in full screen mode but also helped prevent keyboard traps.
Avoiding Keyboard Traps
A common problem encountered on any web page using Flash is tabbing from the HTML elements of the page to the plugin itself. The Flash player plugin is independent of the tab ordering of the page in which it is embedded, and so the user runs the risk of becoming keyboard trapped inside the Flash movie. This problem is
βThe problem is that, while both the Flash content and the HTML content around it may be keyboard accessible, many browsers do not support moving keyboard focus between the Flash content and HTML content without using a mouse. Once focus is placed inside the Flash content, a keyboard user will be trapped there. Similarly, when focus is placed somewhere else on the HTML content (outside the Flash content), it will be impossible to move focus into the content.β
The workaround for this issue is to provide two anchor points either side of the Flash movie that Flash is aware of and can manually pass focus to and from.
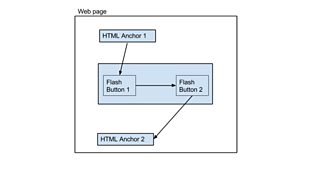
Tab ordering between HTML and Flash
Tab ordering between HTML and Flash
This can be achieved relatively easy using a combination of JQuery and Flash ExternalInterface calls.
The reverse can also be achieved when tabbing backwards from a HTML element to the Flash movie.
.
Screen Reader and Assistive Technology Support
Screen readers are technologies that βscrapeβ a user interface or web page and attempt to convert display elements into another output medium, the most obvious ones being braille and text-to-speech. For HTML based web pages this is a relatively simple task, a screen reader can identify each component based on its HTML tag, an input field, a combo box etc, and can interpret what to do with it. With Flash based components a screen reader is normally unable to interpret the function of a display object and users can become lost when navigating within the movie.
When coding components for screen readers a few basic rules must be followed:
1. any interactive object must have an audio tooltip or alternative text, usually the name of the control
2. the control must be descriptive enough to describe its function, is it a button, slider etc
3. the control must have a description on how to operate it, e.g. press βEnter or Space to activateβ
4. the screen reader must be notified when a component updates or appears, e.g a buffering spinner appears or a component is focused
Microsoft Active Accessibility (MSAA)
Assistive technology adds capabilities that the computer doesn't usually have. For example, a visually impaired user might employ assistive technology such as a screen reader and voice recognition, rather than directly using the application with the mouse and screen. The Microsoft Active Accessibility API was first introduced to Windows as a means of allowing assistive technologies access to control and read applications as well as non-standard UI components. It provides a method for identifying and manipulating user interface controls through non-standard means and allows the same components to alert the system when they change state, e.g. being focused or clicked. Later versions of Flash Player added support for the MSAA spec.
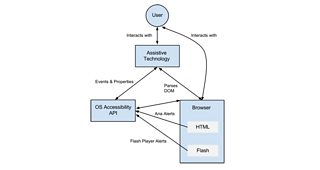
Interactions between the user and the assistive technology interface
The MSAA API allows user controls or visual components to be assigned specific roles; these help the accessibility API to determine what the control does, its current state and how any assistive technologies can best interact with it. For example, a user control that can be clicked can assign itself the role of ROLE_SYSTEM_PUSHBUTTON to inform any accessibility tools that when this component is focused it can be clicked on. More complicated roles include ROLE_SYSTEM_SLIDER which identifies the component as a slider that can be controlled by incrementing and decrementing values.
For a list of the most common MSAA roles .
Whilst this Flash implementation is based on the MSAA spec there are newer accessibility APIs including IAccessible2 which is used in Firefox and Open Office and Microsoft UI Automation, the successor to MSAA.
Adding Alternative Text to Flash Components
The first step in making a Flash component accessible is to tag it with the necessary name and description, when the component becomes focused it will inform the screen reader of its descriptive name and how to operate it.
Flash provides this functionality as part of the .
Initialising the Accessibility API
Interacting with the screen reader is done via .
When a web page is loaded, a screen reader will take a snapshot of the page and maintain it in a virtual buffer, this needs to be kept in sync when the page changes. For static HTML a screen reader can simply traverse the DOM but in Flash we must manually call back to the screen reader to inform it when the movie updates. This call need only be sent once to prevent spamming the screen reader with alerts, normally after all UI components have been initialised.
Accessibility Implementations
As mentioned above, Flash conforms to the MSAA spec which allows display objects to be tagged with specific roles, in Flash this can be done by the .
By tagging a component with an accessibility implementation a screen reader can query it to determine its function, its current state and its default action when activated.
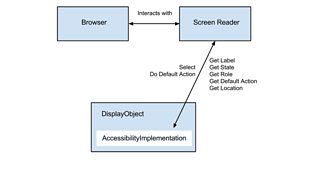
Interaction between a screen reader and an MSAA component
The AccessibleButtonImplementation.as in the project link below is an example accessibility implementation for a push button, it defines how the component operates when focused, its default action and the command to run when that action is triggered by the screen reader.
The button implementation is a very basic example of what can be achieved but shows that you can cater the functionality of a Flash component to better suit the needs of an accessible user if required. You should avoid overcomplicating an interface, avoid nesting accessible components or controls with too many operations, sticking to simple buttons and form elements makes for a better experience and quicker navigation via the keyboard.
For more example accessibility implementations see the Adobe Open Source repo.
Sending Screen Reader Alerts
Occasionally you may wish to inform a screen reader that an operation has taken place without prior user interaction, in the case of a media player this could mean a buffering spinner or error message has been displayed. To avoid confusing the user as to why playback has been interrupted we want to send an audible alert to the screen reader. This can be easily achieved by taking advantage of screen reader support for HTML, by adding a Flash ExternalInterface callback to the webpage we can dynamically create hidden HTML alerts which are detected and read aloud by the screen reader.
Example Code
A working example of all of the concepts discussed, or try out our full accessibility implementation using a screen reader on the
Supported Audio Screen Readers
Based on our research and testing we have determined that the best supported screen readers for Flash Player are Jaws and NVDA . However there are many other products available including Microsoft Narrator, Nokia Talks, WindowEyes, ZoomText and Supernova, all have varying levels of support for Flash and should be considered when testing.
Due to Flash Playerβs implementation of MSAA at the time of writing we only fully support Flash accessibility on Windows devices using Internet Explorer or Firefox. Support for Macintosh users with VoiceOver enabled is unfortunately not available as VoiceOver support for Flash was never implemented and Adobe recently announced their intention to scrap development of an accessible Flash Player for Macintosh. Screen reader support for Flash Player in the Google Chrome browser is also problematic as it bundles its own custom PepperFlash plugin which is incompatible with current screen reader implementations.
The Future
Developing accessible services is a constant, iterative process of user testing and playing catch-up with browser and Flash Player updates. As browser based technologies move on, new standards and alternatives to Flash become more widespread the Standard Media Player will continue to evolve to support them. The mobile and touch screen space also continues to expand - with the launch of services such as Chromecast we are able to investigate alternative user experiences that go beyond sitting in front of PC which may better suit accessible users.
You can read more about our efforts to bring accessibility to SMP and ΒιΆΉΤΌΕΔ iPlayer in .
Due to the specialist requirements that go with building accessible services we do encourage users of assistive technologies and those with other requirements to get in touch to help us develop services further - weβd love to hear your thoughts in the comments below or via the Accessibility email.
Dan Oades is a Senior Software Engineer, Media Playout Team, ΒιΆΉΤΌΕΔ Future Media